Tag Editor Field using jQuery similar to StackOverflow
Sep 9, 2011 • Chris Pietschmann • jQueryThe tag editor featured on the StackOverflow website (and all the StackExchange websites) is a real slick tag editor that makes it much nicer to enter tags than just a plain textbox. I searched a little and couldn’t find anything that works just like theirs, so I decided to build one. At least this was the case back in 2011 when this was originally posted.
The jquery.selectit
Tag editor I built works and look similar to the Tag editor on the StackOverflow website. I hope you find it useful.
You can view the full source code for this here: https://github.com/crpietschmann/jquery.selectit
Here’s the source code for the above usage example of the jquery.selectit
Tag editor:
<div id='SelectBox' style='width: 350px'></div>
<input id='btnClear' type='button' value='Clear' />
<input id='btnGetTags' type='button' value='Get Tags' />
<input id='btnAdd' type='button' value='Add Tag' />
<script src="https://code.jquery.com/jquery-3.7.1.js"></script>
<script src="https://cdn.jsdelivr.net/gh/crpietschmann/jquery.selectit@v1.0/dist/js/jquery.selectit.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/gh/crpietschmann/jquery.selectit@v1.0/dist/css/jquery.selectit.css">
<script>
$(function () {
// initialize tag editor and add a couple
// pre-selected tags
$('#SelectBox').selectit({
// the name of hidden input elements created
fieldname: 'tags',
// pre-selected tags
values: [
'javascript',
'css',
'jquery'
]
});
$('#btnGetTags').click(function () {
// display entered tags to user
alert($('#SelectBox').selectit('values').join(', '));
});
$('#btnClear').click(function () {
// clear al tags from editor
$('#SelectBox').selectit('clear');
});
$('#btnAdd').click(function () {
// programatically add tag to editor
var tag = prompt('Enter tag to add:', '');
if (tag && tag !== '') {
$('#SelectBox').selectit('add', tag);
}
});
});
</script>
Have fun!
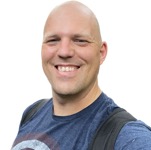
Chris Pietschmann
DevOps & AI Architect | Microsoft MVP | HashiCorp Ambassador | MCT | Developer | Author
I am a DevOps & AI Architect, developer, trainer and author. I have nearly 25 years of experience in the Software Development industry that includes working as a Consultant and Trainer in a wide array of different industries.